- Date
My Rust Programming Journey: Part 1 - Getting started
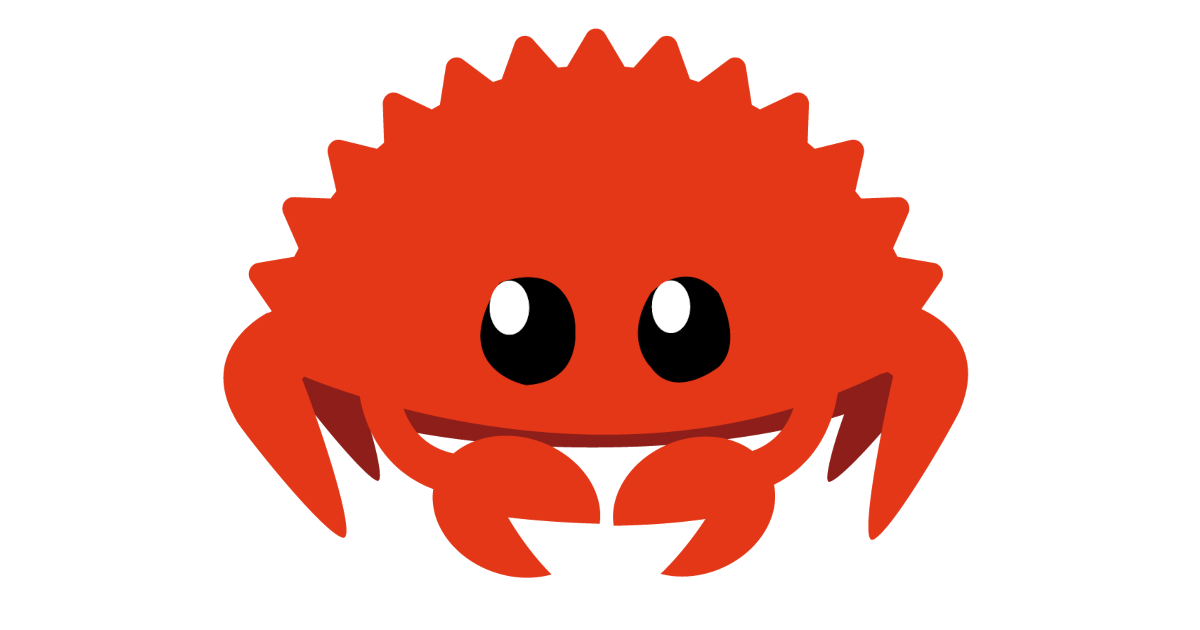
A week ago I started learning the Rust Programming Language.
I was just exploring to see what it’s like to write code in Rust and I ended up considering it for some of my future projects. It has some cool features that make me think of Python and some others that are totally out of this world! So I decided to write a series of posts to share my thoughts on the language.
In the first part of the series, I will just go through the installation of Rust and the creation of a new Rust project. No prior experience in programming is required to follow along.
Getting started with Rust
Let’s get started with the Rust Programming Language. We will install Rust and write a simple program that prints something in the console.
To install Rust on Windows, head over to https://www.rust-lang.org/learn/get-started and click on the download rust-init.exe button corresponding to your operating system’s architecture (32-bit or 64-bit). Run the downloaded file when it completes and just follow the instructions. If you are on a Unix-like system (Mac OS, Debian, Ubuntu…), run the following command in your terminal:
This command will download and run
You should now have Rust installed on your computer. To check that, start a new terminal window and run the following command:
If the installation was successful, you should see the version of Cargo in your terminal. Something like this:
With Rust and Cargo installed, we can create our first Rust project. With your terminal, navigate to the folder where you want to create the project and run the command below:
This will generate a new folder called
What this code does is define a new function called
You should have an output similar to the one below:
You can see that Cargo built our project unoptimized for the dev target and ran the binary that was created (
When you run it, the output should be something like this:
As you can see, Cargo rebuilt the project and ran it. It printed
Conclusion
That was it. We officially created and executed our first program using the Rust Programming Language. It’s true it just prints a sentence in the console but it is possible to get from there to writing fairly complex programs with Rust very quickly. To get there, I will be going through the book which is the official documentation of the Rust Programming Language. I will also be sharing about my Rust journey here. If you don’t want to miss updates, follow me on my social accounts.